How Python Prevents Us from Adding a List as a Dictionary's Key?
Exploring the technical reason.
We all know that Python raises an error when we add a list as a dictionary’s key:
But have you ever wondered how exactly this is implemented in practice? How Python prevents us from adding a list as a dictionary’s key?
This is important to know because in Python, if we define a custom class, then by default, its objects can be added as a dictionary’s key, as shown below:
This behavior may not be desired at times.
Let’s understand the internal mechanisms today!
First, we must understand that EVERYTHING in Python is an object instantiated from some class:
List, tuple, dictionary, etc. are class objects.
Int, float, and string are class objects.
Functions are class objects.
In fact, even classes are also objects.
Adding an object as a dictionary’s key is like hashing the object.
Thus, whenever we add an object as a dictionary’s key, Python invokes the __hash__()
magic method of that object’s class.
Now, the implementation of __hash__()
magic method for classes of int, tuple, string, etc., allows its objects to be hashable.
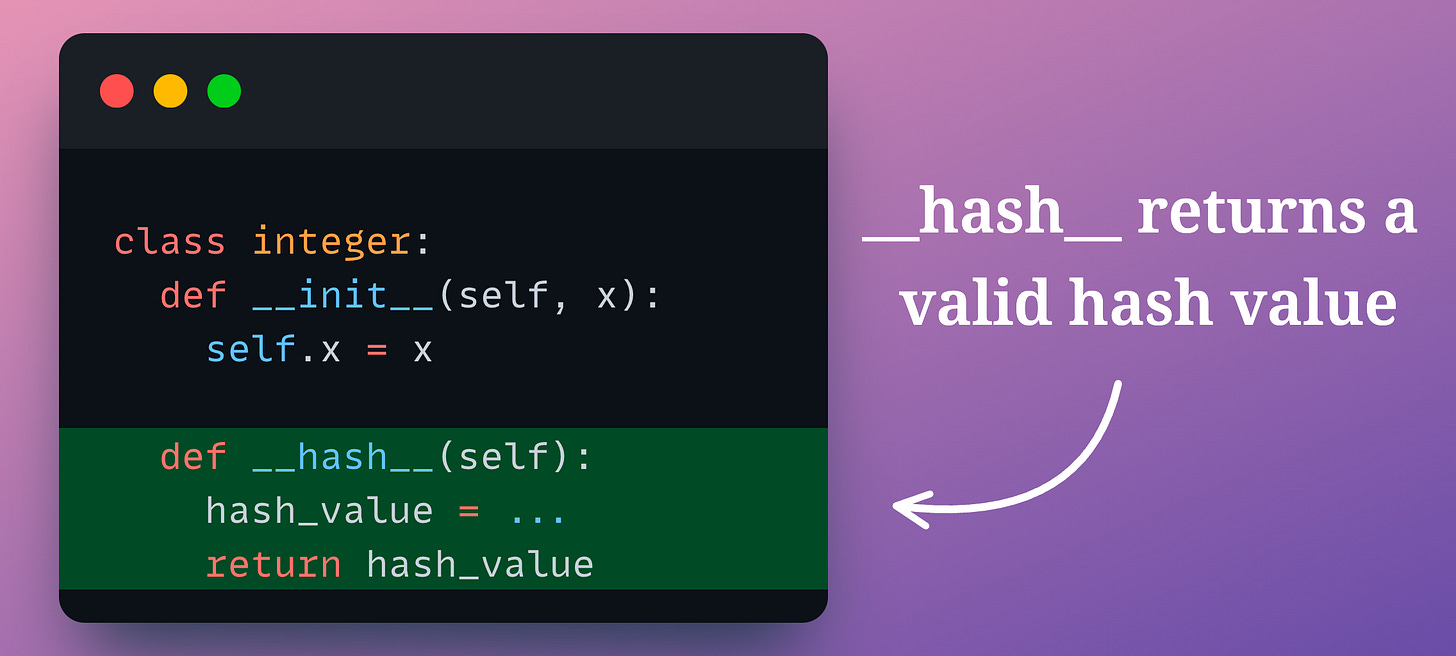
However, the List class raises an error:
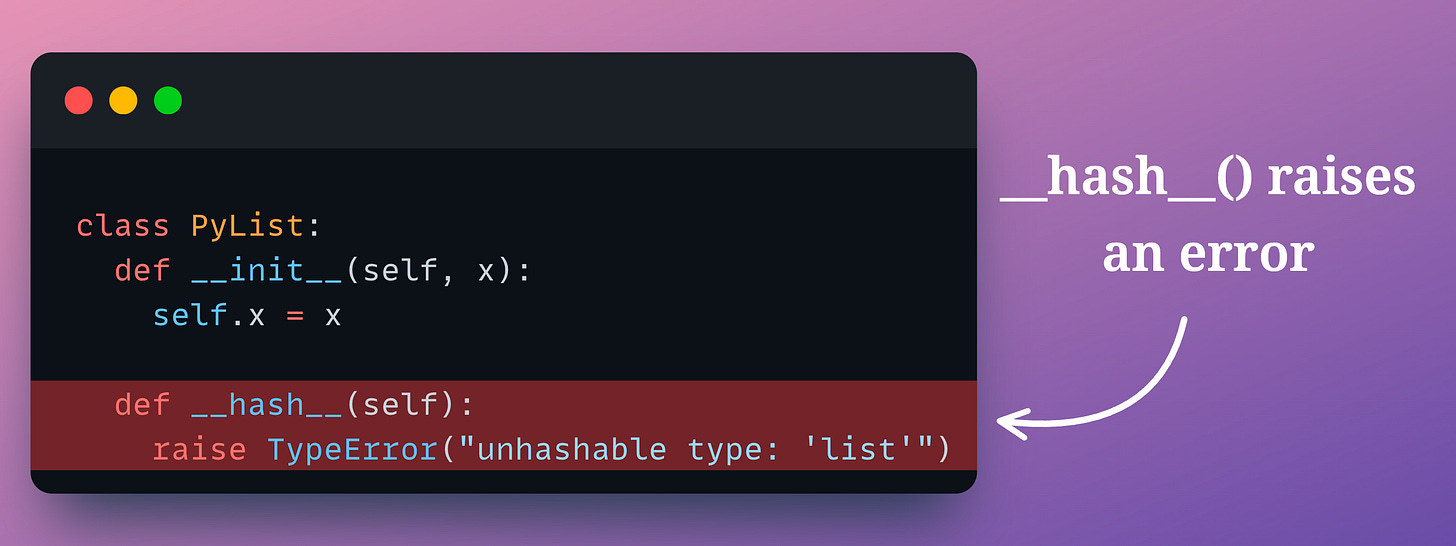
That is why whenever we add a list as a dictionary’s key, Python raises an error:
Thus, if needed, we can use the same idea on our custom classes and make its object unhashable. This is shown below:
Now, if we add its objects to a dictionary, Python will raise an error:
Now you have the complete information (conceptual and practical) on why some objects can be added as a dictionary’s key while some cannot.
Here’s a full deep dive into Python OOP if you want to learn more about advanced OOP in Python: Object-Oriented Programming with Python for Data Scientists.
👉 Over to you: What are some other core yet overlooked concepts in Python?
Thanks for reading!
In case you missed it
I unlocked the following three deep dives recently. Today is the last day that they will remain open.
Model Compression: A Critical Step Towards Efficient Machine Learning → Learn how companies save 1000s of dollars in deployment costs.
You Cannot Build Large Data Projects Until You Learn Data Version Control! → Learn how ML projects are made 100% reproducible with code and data versioning.
Why Bagging is So Ridiculously Effective At Variance Reduction? → Learn the mathematical foundation of Bagging, using which, you can build your own Bagging-based models.
These are a must-read if you want to build core ML skills in a beginner-friendly way.
Are you preparing for ML/DS interviews or want to upskill at your current job?
Every week, I publish in-depth ML deep dives. The topics align with the practical skills that typical ML/DS roles demand.
Join below to unlock all full articles:
Here are some of the top articles:
[FREE] A Beginner-friendly and Comprehensive Deep Dive on Vector Databases.
A Detailed and Beginner-Friendly Introduction to PyTorch Lightning: The Supercharged PyTorch
Don't Stop at Pandas and Sklearn! Get Started with Spark DataFrames and Big Data ML using PySpark.
Federated Learning: A Critical Step Towards Privacy-Preserving Machine Learning.
Sklearn Models are Not Deployment Friendly! Supercharge Them With Tensor Computations.
Deploy, Version Control, and Manage ML Models Right From Your Jupyter Notebook with Modelbit
Join below to unlock all full articles:
👉 If you love reading this newsletter, share it with friends!
👉 Tell the world what makes this newsletter special for you by leaving a review here :)
btw, lists aren't hashable because they're mutable. from so:
When you store the hash of a value in, for example, a dict, if the object changes, the stored hash value won't find out, so it will remain the same. The next time you look up the object, the dictionary will try to look it up by the old hash value, which is not relevant anymore.
To prevent that, python does not allow you to has mutable items.
Nice trick! Python needs immutable and hashable keys to guarantee that lookups are consistent and fast.